Post Contents
What is data structure
The data structure is a way to store and organize data in a computer system. So that we can use the data easily.
To solve a problem, store and organize the data in computer memory in a systematic way so that it can be used efficiently is called a data structure.
That is, the data is stored and organized in such a way that it can be easily accessed later at any time.
As a programmer, you have to solve many real-world problems. Data and its organization are very important when solving these problems.
For example, you use a dictionary to know the true meaning of a word. With Dictionary, you can find any word and its meaning very easily. You can do this because all the words (data) in the dictionary are stored in a systematic way.
If dictionary words are stored randomly instead of storing them in sorted order, it may take you several days to find the meaning of a word. Dictionary is a real-life example of data structure and its importance.
The way words are organized in the dictionary helps in finding the meaning of words quickly and easily. Similarly, there are many problems that you need to store and organize the data in a systematic way to solve it.
As a programmer, you may have to perform the tasks given below to solve problems.
• Create data structures.
• Performing operations (searching, sorting, traversing, etc.) with data structures.
• Analyze the performance of data structures.
In the following tutorials, you will learn to create different types of data structures and perform operations with those data structures. Apart from this, you will also learn to analyze the performances of data structures.
One thing you should always keep in mind is that data structures do not have any language. The data structure is simply a way to store and organize data that can be implemented in any programming language such as C, C ++ and java, etc.
Because C is a basic language whose syntax is mostly followed by modern programming languages. Therefore, in this tutorial series of data structures, we will be told to implement data structures by C language.
Characteristics of Data Structures
Any data structure has the following characteristics.
Correctness
The most important feature of a data structure is that it correctly resolves the problem. If a data structure resolves a problem partially (half-incomplete), then it cannot be considered to be the correct data structure for that problem.
Time complexity
The time complexity that a data structure takes to perform operations is called the time complexity of that data structure. Performance analysis and measurement tools are used to check the time complexity of different data structures.
The operations performed by a data structure take the least time. The data structure by which the operations performed take the least time is considered correct to solve the same data structure problem.
Space complexity
The amount of computer memory space that a data structure uses for storage and operations of data is called space complexity of that data structure. Several performances and analysis tools are also used to test space complexity.
The data that is stored by a data structure and the operations to be performed using the least memory space. The data structure by which the least space is used is considered the best solution to solve the problem.
Advantages of Data Structures
Some advantages of implementing and using data structures are given below.
Gives Ability to Process Data and Store in Desired Way
One of the biggest advantages of data structures is that you can store the data in your desired way and can perform operations on it as you want. You are not obliged to follow a certain framework.
This gives you the freedom to implement creative solutions to solve problems.
Uses Less Processor Time
The operations performed by the data structure use the least time of the processor. This causes less load on the computer processor and it is also able to process a large amount of available data in less time.
Uses Less Processor Time
The operations performed by the data structure use the least time of the processor. This causes less load on the computer processor and it is also able to process a large amount of available data in less time.
Uses Less Memory Space
When you work on a big project, properly utilizing the memory of the computer is a priority. Data structures acquire at least memory space as a solution to a problem. It has enough memory for you to perform other tasks and does not cause problems like memory loss or shortage.
Easy to Perform Complex Operations
Complex operations can be easily used in the context of a problem by data structures.
Types of data structure
Introduction to Data Structure Types
By storing and organizing data in the same way, you cannot solve different problems. To solve different types of problems, you need to create different types of data structures.
Each type of data structure has a different data organization mechanisms and operational behavior which makes it different from other data structures.
For example, some data structures are already provided to you by programming languages and some data structures you create yourself. Some data structures are simple and some data structures are very complex. Some data structures allow operations by the user and some data structures also perform operations automatically.
Data structures are divided into different categories based on similar characteristics. This is shown by the following block diagram.
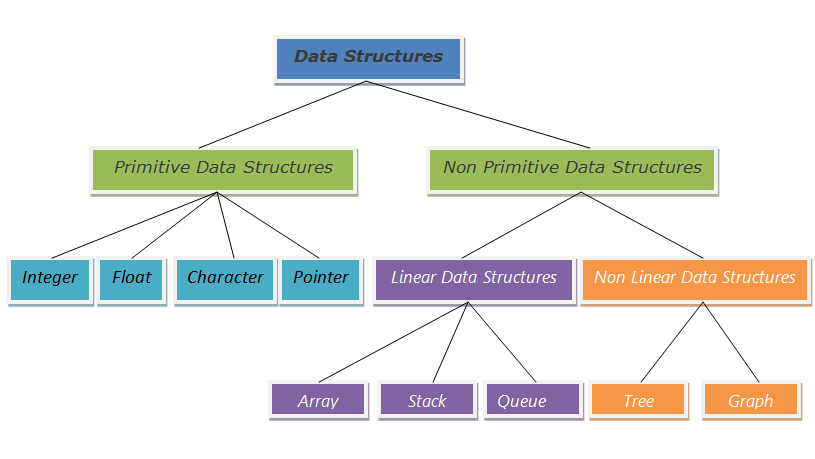
The classification diagram of different types of data structures is shown in the above block diagram. Let us now try to know in detail about different types of data structures.
Primitive Data Structures
A primitive data structure is a data structure that can be directly operated by machine instructions.
That is, it is defined by the system and compiler.
A data type provides a structure to store data of a specific type. Therefore primitive data types provided by programming languages are also considered as data structures. These data structures are called primitive data structures.
All programming languages provide the primitive data types given below.
• int – to store Integer data.
• float – to store floating-point data.
• double – It is similar to data float. It is used to store values greater than 7 after the decimal.
• character – to store words.
• boolean – to store true and false values.
Non-primitive Data Structures
Non-primitive data structure: – The primitive data structure is a data structure that cannot be operated by direct machine instructions.
Types of non-primitive data structure: –
1. Linear data structure
2. Non-linear structure
Non-primitive data structures consist of a combination of the same type or different types of primitive data structures. For example, an array of integer numbers is a non-primitive data structure.
Non-primitive or user-defined data types are created by a combination of primitive data types so user-defined data types are also called non-primitive data structures.
Non-primitive data structures (Linear and Non-linear) are divided into two categories. They are being told below.
1.Linear data structure: –
Linear is a data structure in which data items are stored and organized in linear (linear) form, in which one data item is connected to another as a line.
Linear data structures are data structures that store elements in a linear sequence. For example, the elements of an array contain one store after another at continuous locations.
ex: – array, linked list, queue, stack.
An overview of the linear data structures you will learn about in this tutorial series is given below.
Arrays
An array is the simplest non-primitive linear data structure. Elements in an array are stored in contiguous memory locations. An array is a collection of variables of the same (data) type that is represented by a common name.
For example, you can create an array of floating-point numbers and store floating-point numbers in it.
Stacks
A stack is a linear data structure in which elements are added and removed from the same side (top). Elements in a stack are organized in the same way as plates (one on top) are arranged in a restaurant.
Like the plate placed at the end is raised first. Similarly, the last inserted element in a stack is the first access and the first inserted element is the last access.
Queues
A queue is a data structure in which elements are inserted from one side (from the backside) and removed from the other side (from the front).
Just like when you stand in the last line while standing in a line and go to the forefront after getting service. Similarly, a queue data structure also works in the First In First Out order in which elements are inserted from one side and removed from the other side.
Singly Linked Lists
A linked list data structure is a linear collection of elements. An element in a linked list data structure points to another element. A Next pointer or link node is associated with each element that points to the next element of the list in memory.
Through the linked list, you can overcome the drawbacks of arrays and use a data structure that makes proper utilization of memory and in which operations can be performed easily.
2.Non-linear data structure: –
Non-linear is a data structure in which data items are not arranged in a sequential manner.
In which a data item can be associated with any other data items.
Non-linear data structures are data structures that do not store elements in a linear sequence. For example, elements in a tree data structure are not stored in a linear sequence, so the tree is a non-linear data structure.
ex: -tree, graph.
An overview of the non-linear data structures you will learn about in this tutorial series is given below.
Trees
Tree data structures are used to represent data in which an entity and its attributes have a hierarchical relationship. In the tree data structure, data and its entities are represented as parent nodes and child nodes.
A node points to another node in a linked list but a node in a tree data structure can point to multiple nodes. Child nodes can also be child nodes in the tree data structure.
Graphs
Graphs are non-linear data structures that are used in many ways. Graphs are also used for the analysis of electrical circuits, for finding shortest routes, for project planning, for representing highways, landlines, and railway lines, etc.
Data Structure Operations
Introduction to Data Structures Operations
Data structures are created to perform some important operations. All data structures perform the same operations. But operations are performed differently by every data structure.
For example, in Stack, data is inserted and removed from the same side, whereas in Queue, data is inserted from one side and removed from the other side.
Various data structure operations are used to process data in a data structure which is as follows: –
Traversing
Visiting each element of the data structure only once is called traversing.
In the traversing operation, every record of data is accessed only once so that it can be processed. Traversing operation is performed by loops. For example, you traverse the elements of an array through a loop and get them printed.
Insertion
Adding the same type of element to the data structure is called insertion. An element can be added anywhere in the data structure.
In insertion operation, data insert is done in a data structure. The insertion operation is known by different names in different data structures.
For example in relation to Stack, this operation is called push operation. Whereas in the context of Queue, this operation is called Enqueue operation.
Deletion
Removing an element from a data structure is called Deletion. An element in a data structure can also be removed from anywhere.
The deletion operation is known by different names in different data structures.
For example, in the context of Stack, this operation is called Pop. Whereas in the context of Queue, this operation is called Dequeue.
Searching
Finding an element in the data structure that satisfies one or more conditions.
When a data search is done in a data structure, it is called operation searching. This is a very important operation which is performed frequently.
Many different techniques are used to perform searching operations which are called searching techniques. For example, a linear search and binary search are search techniques.
Sorting
Sorting is to arrange the elements in the data structure in ascending and descending order.
Arranging data in a certain order is called sorting. For example, if data is text, it is sorted in alphabetical order (a – b – c .. etc.). If data is numeric, it is sorted in ascending (1 -2 -3 ..n) or descending (n… 3 – 2 -1) order.
Different types of techniques are used to perform sorting operations, which are called sorting techniques. For example selection sort, bubble sort and insertion sort, etc. are the major sorting techniques.
Merging
Merging is called combining and storing elements in two different data files in one data file.
In the merging operation, two data structure records are combined as a single record. This operation is also performed with a sorting operation.
Some genuinely interesting details you have written.Assisted me a lot, just what I was looking for : D.